Crypto currency markets are open 24/7, automated trading makes it a valuable tool in your boots. In this article, the Python-Binance using the library, we will tell you step by step how to improve a simple but effective trading boat.
- Installation and preparation necessary
First, we need to install the necessary libraries:
pip install python-binance
pip install pandas
pip install numpy
Binance API keys create:
- Binance log in to your account
- Go to the section API management
- Create the API key, click e
- API key and Secret Keyand then store it in a safe place
from binance.client import Client
from binance.enums import *
import pandas as pd
import numpy as np
import time
# API bilgilerini güvenli bir şekilde saklayın
api_key = 'YOUR_API_KEY'
api_secret = 'YOUR_API_SECRET'
# Binance client'ı başlatma
client = Client(api_key, api_secret)
- Basic data retrieval and processing
def get_historical_data(symbol, interval, lookback):
"""
Geçmiş fiyat verilerini çeker
Parametreler:
symbol (str): Trading çifti (örn: 'BTCUSDT')
interval (str): Zaman aralığı (örn: '1h', '15m')
lookback (str): Ne kadarlık geçmiş veri
"""
try:
df = pd.DataFrame(client.get_historical_klines(
symbol,
interval,
lookback + " ago UTC"
))
df = df.iloc[:, :6]
df.columns = ['Time', 'Open', 'High', 'Low', 'Close', 'Volume']
df = df.set_index('Time')
df.index = pd.to_datetime(df.index, unit='ms')
df = df.astype(float)
return df
except Exception as e:
print(f"Veri çekme hatası: {e}")
return None
- Creating A Simple Trading Strategy
As an example, moving average, moving average) Crossover strategy, we use:
def calculate_signals(df, short_window=20, long_window=50):
"""
Trading sinyalleri oluşturur
"""
df['Short_MA'] = df['Close'].rolling(window=short_window).mean()
df['Long_MA'] = df['Close'].rolling(window=long_window).mean()
df['Signal'] = 0
df.loc[df['Short_MA'] > df['Long_MA'], 'Signal'] = 1
df.loc[df['Short_MA'] < df['Long_MA'], 'Signal'] = -1
return df
def execute_trade(symbol, side, quantity, price):
"""
Trade işlemini gerçekleştirir
"""
try:
if side == "BUY":
order = client.create_order(
symbol=symbol,
side=SIDE_BUY,
type=ORDER_TYPE_LIMIT,
timeInForce=TIME_IN_FORCE_GTC,
quantity=quantity,
price=str(price)
)
else:
order = client.create_order(
symbol=symbol,
side=SIDE_SELL,
type=ORDER_TYPE_LIMIT,
timeInForce=TIME_IN_FORCE_GTC,
quantity=quantity,
price=str(price)
)
return order
except Exception as e:
print(f"Trade hatası: {e}")
return None
- Class Main Trading Bot
class TradingBot:
def __init__(self, symbol, interval, lookback):
self.symbol = symbol
self.interval = interval
self.lookback = lookback
self.position = False
def run(self):
while True:
try:
# Verileri al
df = get_historical_data(self.symbol, self.interval, self.lookback)
if df is None:
continue
# Sinyalleri hesapla
df = calculate_signals(df)
# Son sinyali al
current_signal = df['Signal'].iloc[-1]
current_price = float(df['Close'].iloc[-1])
# Trading mantığı
if current_signal == 1 and not self.position:
# Alış sinyali
quantity = self.calculate_position_size(current_price)
order = execute_trade(self.symbol, "BUY", quantity, current_price)
if order:
self.position = True
print(f"ALIŞ: {quantity} {self.symbol} @ {current_price}")
elif current_signal == -1 and self.position:
# Satış sinyali
quantity = self.get_position_quantity()
order = execute_trade(self.symbol, "SELL", quantity, current_price)
if order:
self.position = False
print(f"SATIŞ: {quantity} {self.symbol} @ {current_price}")
# Bekleme süresi
time.sleep(60) # 1 dakika bekle
except Exception as e:
print(f"Bot çalışma hatası: {e}")
time.sleep(60)
def calculate_position_size(self, price):
"""
Risk yönetimi ve pozisyon büyüklüğü hesaplama
"""
balance = float(client.get_asset_balance(asset='USDT')['free'])
risk_percentage = 0.02 # %2 risk
position_size = (balance * risk_percentage) / price
return round(position_size, 6)
def get_position_quantity(self):
"""
Mevcut pozisyon miktarını öğrenme
"""
balance = float(client.get_asset_balance(asset=self.symbol.replace('USDT', ''))['free'])
return round(balance, 6)
- Run The Boat
if __name__ == "__main__":
# Bot parametreleri
symbol = "BTCUSDT"
interval = "15m"
lookback = "1 day"
# Botu başlat
bot = TradingBot(symbol, interval, lookback)
bot.run()
Important notes and safety precautions:
- Risk Management
- Always test with a small amount
- Use stop-loss
- Determine your risk management strategy
- Security Measures
- Keep your API keys secure
- Use IP restrictions
- Just give trading permissions
- Testing
- The first test network (testnet) try
- On paper (paper trading) test
- Living with a small amount go to
- Monitoring and logging
import logging
logging.basicConfig(
filename='trading_bot.log',
format='%(asctime)s - %(levelname)s - %(message)s',
level=logging.INFO
)
These code samples and explanations, basic can be a starting point to develop a trading boat. But remember, your strategy should be thoroughly tested before trading with real money and you must be careful about risk management.
Also, this example uses a simple strategy. More complex strategies, indicators, and risk management techniques you can develop by adding the boat. Always do the process, by considering market conditions, and legal aspects.
By developing your own strategies and by adding the code examples in this article to suit your needs, you can create a trading bot. Good luck!
Boat advanced trading features and tips
Some of the advanced features that you can add to make your boat more efficient Trading:
- The Integration Of The Telegram
import telegram
def send_telegram_message(bot_token, chat_id, message):
bot = telegram.Bot(token=bot_token)
bot.send_message(chat_id=chat_id, text=message)
- Multiple Time Frame Analysis
def multi_timeframe_analysis(symbol):
timeframes = ['1h', '4h', '1d']
signals = {}
for tf in timeframes:
df = get_historical_data(symbol, tf, "1 week")
signals[tf] = calculate_signals(df)
return signals
- Volatility-Based Risk Management
def calculate_volatility(df):
return df['Close'].pct_change().std()
def adjust_position_size(price, volatility):
base_size = calculate_position_size(price)
return base_size * (1 - volatility)
These additional features, your boots will ensure the operation more efficient and secure. Also, by doing a regular backtest your strategy to evaluate the performance of Remember.
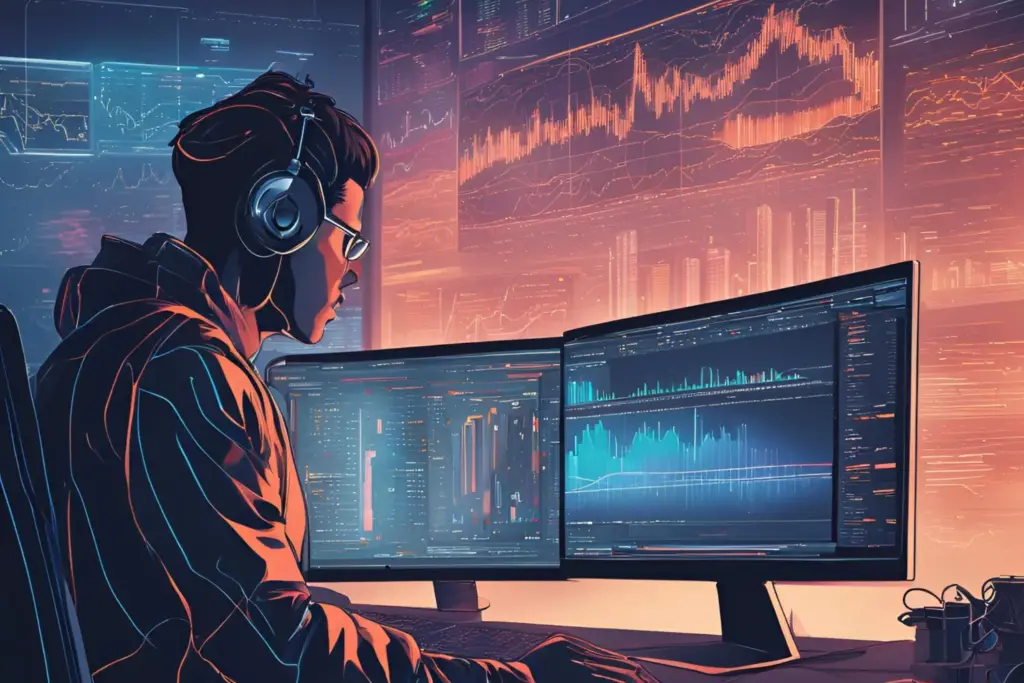